Visual Studio Code Extension Samples
Hi Michael Shpilt, There is no words to praise your hard efforts and well presented series on Visual studio Extension development. Finding a good information on professional scale code samples on Extension development on internet is very difficult. Mostly we will find documentation or some very basic hello world kind of samples. While the Extension Capabilities section offers high-level overviews of what an extension can do, this section contains a list of detailed code guides and samples that explains how to use a specific VS Code API. In each guide or sample, you can expect to find: Thoroughly commented source code.
Tutorial
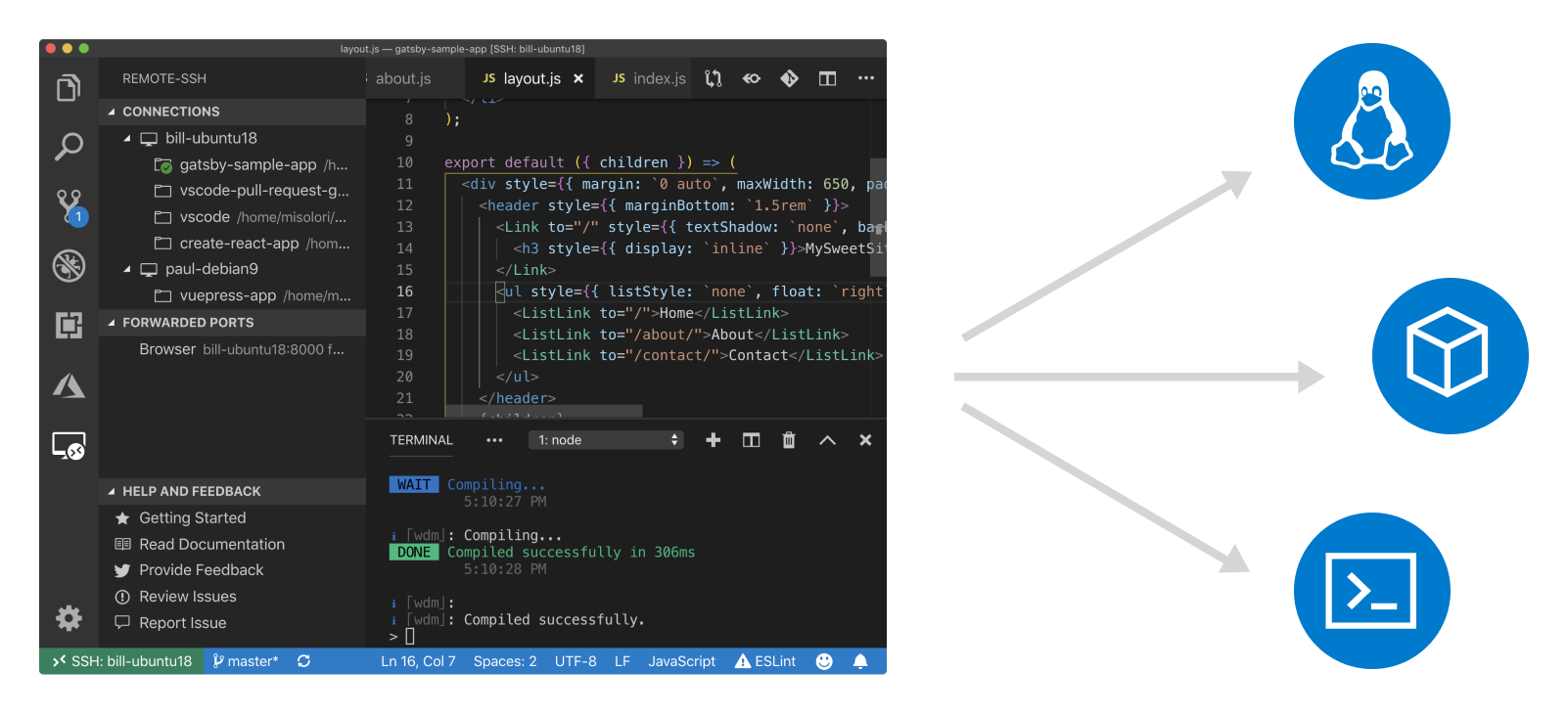
Download Visual Studio Code Install the Salesforce Extensions. Code Builder (coming soon) Code Builder is a browser-based version of Salesforce Extensions, with everything installed and pre-configured for you. It’s provides all the goodness of the desktop experience, but provides you the flexibility to work anywhere, from any computer.
While this tutorial has content that we believe is of great benefit to our community, we have not yet tested or edited it to ensure you have an error-free learning experience. It's on our list, and we're working on it! You can help us out by using the 'report an issue' button at the bottom of the tutorial.
Introduction
Visual Studio Code is a code editor from Microsoft available on Windows, Linux, and macOS. It offers extensions that you can install through the Visual Studio Code MarketPlace for additional features in your editor. When you can’t find an extension that does exactly what you need, it is possible to create your own. In this article, you’ll create your first Visual Studio Code extension.
Prerequisites
- Node.js installed on your machine following How To Install Node.js and Create a Local Development Environment.
Installing the Tools
The Visual Studio Code team created a generator for creating extensions, which generates all of the necessary starter files to begin creating your extension.
To get started, you’ll need to have Yeoman installed, which is a scaffolding tool. You can install Yeoman by running
With Yeoman installed, now you need to install the specific generator for Visual Studio Code extensions:
Creating Your First Extension
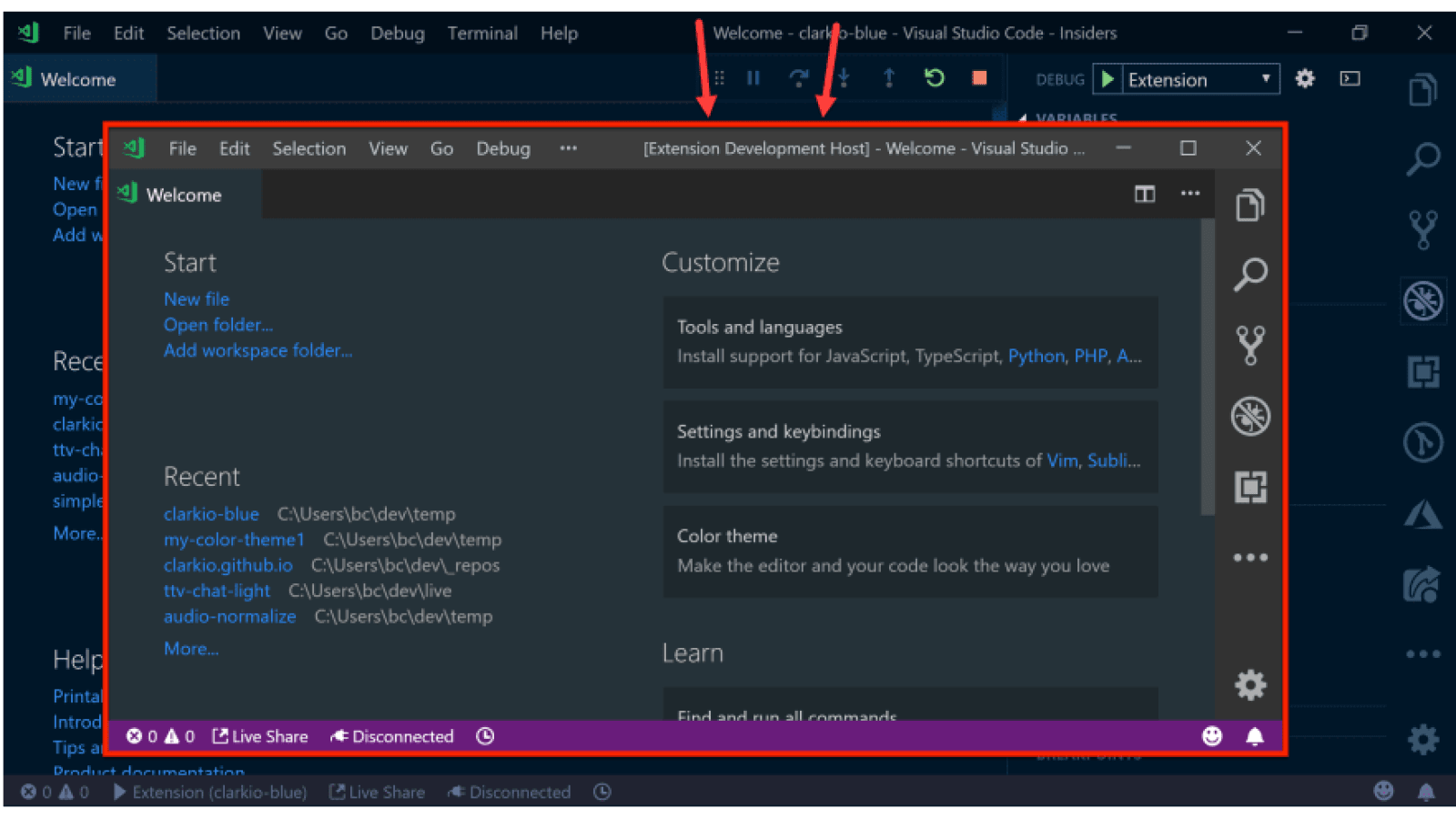
You are now ready to create your first extension. To do so, run the following command.
You will then answer several questions about your project. You will need to choose what kind of extension you are creating and between TypeScript and JavaScript. We will be choosing JavaScript in this tutorial.
Then you’ve got a few more questions.
- name
- identifier
- description
- type checking (yes)
- do you want to initialize a git repository (yes)
After this process is complete, you’ve got all of the files you need to get started. Your two most important files are:
package.json
extension.js
Open package.json
and let’s take a look. You’ll see the name, description, and so on. There are two more sections that are very important.
activationEvents
: this is a list of events that will activate your extension. Extensions are lazy loaded so they aren’t activated until one of these activation events occur.commands
: list of commands that you provide the user to run via your extension.
We will come back to these shortly.
You can also take a look at the extension.js
file. This is where we are going to write the code for our extension. There’s some boilerplate code in here, so let’s break it down.
In the highlighted line below is where our command is being registered with VS Code. Notice that this name extension.helloworld
is the same as the command in package.json
. This is intentional. The package.json
defines what commands are available to the user, but the extension.js
file registers the code for that command.
In this Hello World example, all this command will do is display a Hello World message to the user.
Debugging Your Extension
Now that we have all of the necessary files installed, we can run our extension.
The .vscode
folder is where VS Code stores configuration files of sorts for your project. In this case it includes a launch.json
that contains debug configurations.
From here, we can debug. Open the debug tab on the left on the left of your screen, and then click play.
This will open up a new (debug) instance of VS Code.
With this debug instance of VS Code open, you can open the command palette with Cmd + SHIFT + P
on Mac or CTRL + SHIFT + P
on Windows and run 'Hello world'
.
You’ll see a hello world message pop up in the lower right hand corner.
Editing Your Extension
Top Visual Studio Code Extensions
Before we work on code, let’s take one more look at the activationEvents
section in the package.json
file. Again, this section contains a list of events that will activate our extension whenever they occur. By default, it is set to activate when our command is run.
In theory, this event could be anything, and more specifically *
anything. By setting the activation event to *
this means your extension will be loaded when VS Code starts up. This is not required by any means, just a note.
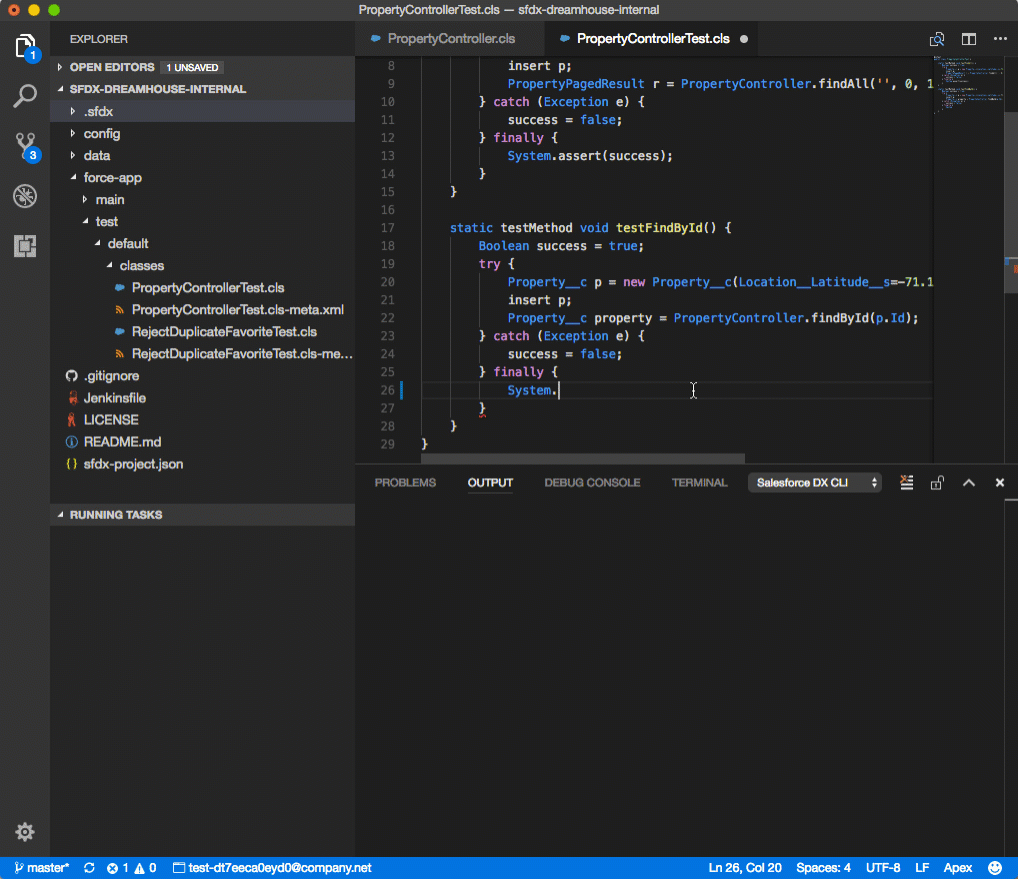
We’ve got the necessary files and we know how to debug. Now let’s start building our extension. Let’s say we want this extension to be able to create an HTML file that already has boilerplate code in it and is added into our project.
Let’s first update the name of our command. In extension.js
, update the name of the command from extension.helloworld
to extension.createBoilerplate
.
Now, update the package.json
file accordingly witht he change in command.
Visual Studio Code Extension Development
Now, let’s write our functionality. The first thing we’ll do is require a couple of packages. We are going to use the fs
(file system) and path
modules.
Visual Studio Code Extension Samples Template
We also need to get the path to the current folder. Inside of the command, add the following snippet:
We will also need to store our boilerplate HTML code into a variable so that we can write that to a file. Here’s the boilerplate HTML:
Now we need to write to the file. We can call the writeFile
function of the file system module and pass in the folder path and HTML content.

Notice that we use the path module to combine the folder path with the name of the file we want to create. Then inside of callback, if there is an error, we display that to the user. Otherwise, we let the user know that we created the boilerplate file successfully:
Here’s what the full function looks like:
Go ahead and debug your newly developed extension. Then, open up the command palette and run “Create Boilerplate” (remember we changed the name).
After running the command, you’ll see the newly generated index.html
file and a message to let the user know:
Conclusion
To learn more about what APIs there are to use and how to use them, read through the Visual Studio Code Extension API documentation.
As I explained in the post Some implications of the new modular setup of Visual Studio 2017 for VSX developers, Visual Studio 2017 has changed all that you knew about installations of Visual Studio. In this episode of Channel 9, Art Leonard explains to Robert Green the internals of this re-architecture of Visual Studio:
The use of a private registry file causes that if you want to know programmatically the installed editions of Visual Studio 2017, the old approaches don’t work. For example, my article HOWTO: Detect installed Visual Studio editions, packages or service packs is now obsolete.
Fortunately, Microsoft provides a new Setup API to query the installed editions of Visual Studio 2017 or the highest VSIXInstaller.exe, along with sample code and utilities:
Visual Studio Setup Configuration Samples
Microsoft/vs-setup-samples
“This is a sample in various programming languages that demonstrates how developers can use the new Visual Studio setup query API. The included samples show how to use the new setup configuration API for discovering instances of Visual Studio 2017”.
Visual Studio Locator
Microsoft/vswhere
“Over the years Visual Studio could be discovered using registry keys, but with recent changes to the deployment and extensibility models a new method is needed to discover possibly more than once installed instance. These changes facilitate a smaller, faster default install complimented by on-demand install of other workloads and components. vswhere is designed to be a redistributable, single-file executable that can be used in build or deployment scripts to find where Visual Studio – or other products in the Visual Studio family – is located.”
Visual Studio Setup PowerShell Module
Microsoft/vssetup.powershell
“This PowerShell module contains cmdlets to query instances of Visual Studio 2017 and newer. It also serves as a more useful sample of using the Setup Configuration APIs than the previously published samples though those also have samples using VB and VC++.”
VSIX Installer Bootstrapper
Microsoft/vsixbootstrapper
“An installer that can be chained with other packages to locate the latest VSIXInstaller.exe to use for installing VSIX extensions. One of the great new features of Visual Studio 2017 is an initial smaller and fast install. To compliment a smaller – but powerful – initial feature set, installing additional workloads and components on-demand is supported for both end users and package developers. Package developers can install their VSIX extensions for Visual Studio using this bootstrapper to find the latest version of VSIXInstaller.exe and install their extension(s). This may be preferable for extensions that support Visual Studio 2017 or newer than installing extensions in Windows Installer .msi packages, since MSIs cannot run concurrently in separate processes. Other deployments may also benefit since they no longer have to find where VSIXInstaller.exe is installed. The command line passed to VSIXBootstrapper.exe is passed through to VSIXInstaller.exe.”